Boolean Best Practices¶
It is best to use booleans in your scripts rather than using an integer to check for the truth of a statement. The reason for this is that it cleans up the code and makes it more intuitive when mapping values to parameter.
For example, if a parameter is called Create Full Backup, true
makes more
sense than 1
Bash¶
true
and false
are native to Mac and Linux operating systems,
this means variable can be evaluated without assigning integers.
For example:
1 2 3 4 | if {attuneVar} then echo "true" fi |
Attune substitutes the a value into the {attuneVar}
parameter.
If the value is true
:
1 2 3 4 | if true then echo "true" fi |

If you want to evaluate a variable as false, you can use the NOT operator !
1 2 3 4 | if ! false then echo 'This is false' fi |
Multiple values can be evaluated with the AND operator &&
1 2 3 4 | if true && ! false then echo 'The first value is true and the second value is false' fi |
Powershell¶
The same is true for Powershell except Powershell use $true
and $false
.
For example:
1 2 3 | if (${{attuneVal}}) { Write-Host "true" } |
Attune substitutes the value into the {attuneVar}
parameter.
If the value is true
:
1 2 3 | if ($true) { Write-Host "true" } |
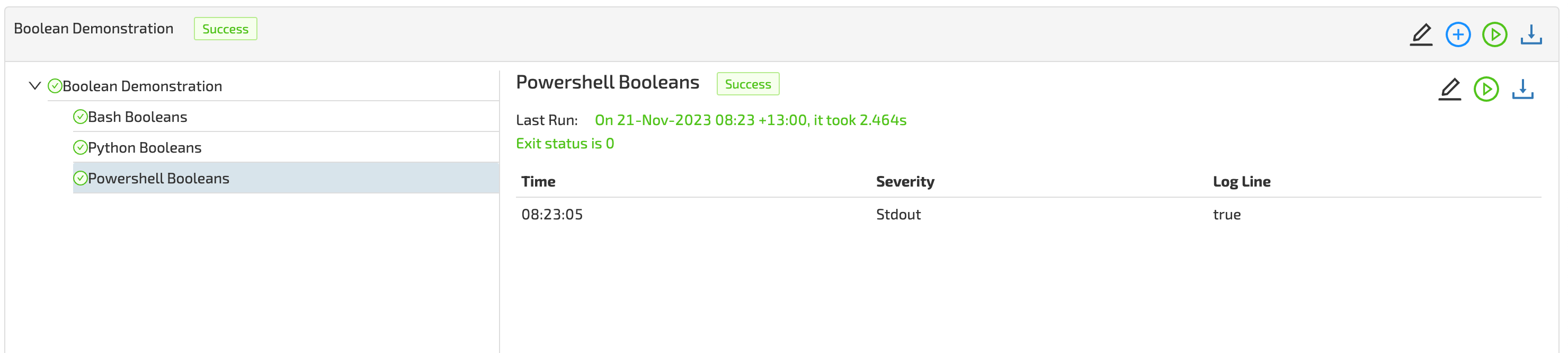
If you want to evaluate a variable as false, you can use the NOT operator -not
1 2 3 | if -not ($false) { Write-Host "Not False" } |
Python¶
Python uses True
and False
. To keep the use of booleans consistent
throughout a project, you are able to assign the booleans to variables at the
beginning of a Python script.
For example:
1 2 3 4 5 6 7 | true=True false=False if {attuneVal}: print("Do the work for true") else print("Do the work for else") |
If the Parameter is mapped to true
, Attune substitutes true
into the {attuneVal}
parameter
which is assigned to True
.
1 2 3 4 5 6 7 | true=True false=False if true: print("Do the work for true") else print("Do the work for else") |
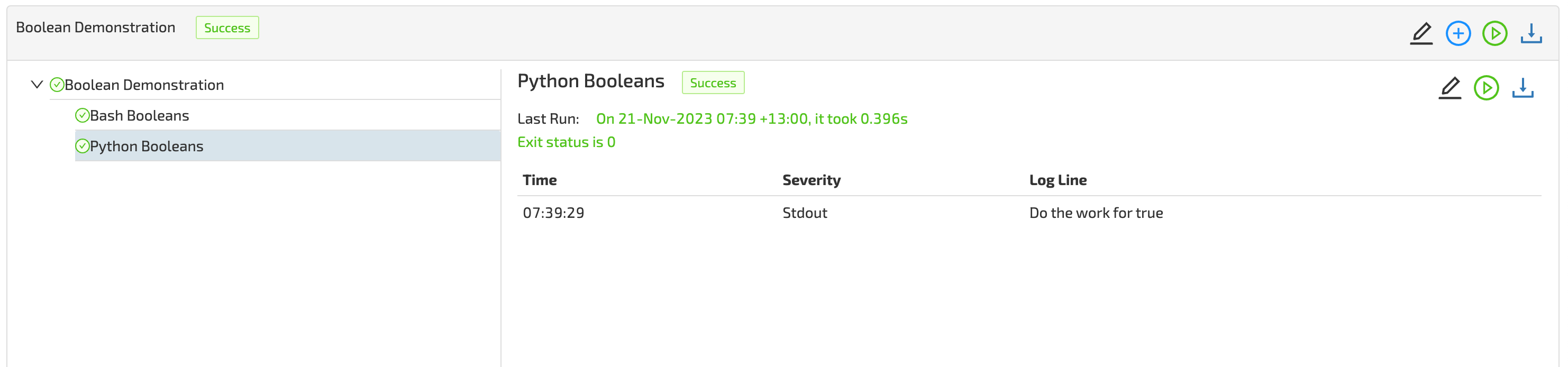
If you want to evaluate a variable as false, you can use the NOT operator not
1 2 3 4 5 6 7 | true=True false=False if not false: print("Do the work for true") else print("Do the work for else") |
Multiple values can be evaluated with the AND operator and
1 2 3 4 5 6 7 | true=True false=False if not false and true: print("Do the work for true") else print("Do the work for else") |